Creating a WordPress post using Python is surprisingly easy.
But when I was learning how to do this, I found that the online examples were either too complex or too simple.
I didn’t want a complex authentication method that required installing a plugin. And I needed more from an example than a one-line post.
Here is the simplest example of a realistic WordPress post using the python-wordpress-xmlrpc library.
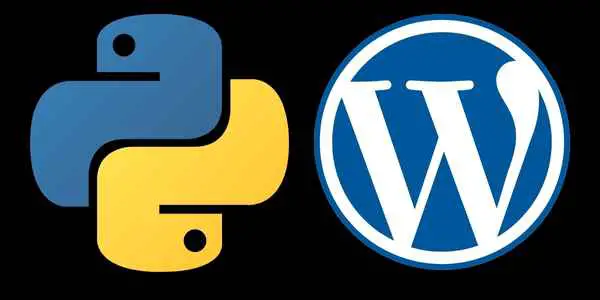
Table of Contents
Python Library For Creating WordPress Posts
You only need to install one library: python-wordpress-xmlrpc.
There are several options to install it. If you are using the command line, this command will download and configure the library in your local environment:
pip install python-wordpress-xmlrpc
Importing The Required Classes From wordpress-xmlrpc
At the top of your Python script, you need to import several classes from the xmlrpc library:
from wordpress_xmlrpc import Client, WordPressPost
from wordpress_xmlrpc.methods.posts import NewPost
from wordpress_xmlrpc.methods import posts
How To Log Into Your WordPress Website
In order to write posts via Python, your script needs to log in and be authenticated.
There are several secure ways to do this that are recommended if you are running your script on a server. They include installing an authentication plugin.
As I was running my script locally against a test website, I didn’t go to these lengths. You can pass a username and password for your WordPress website straight from your script.
I should mention that this isn’t very secure, but it’s certainly the easiest way to get started.
The single line below will log into your website and lets you access posts and other WordPress features. Substitute these items:
- Your domain name
- WordPress login
- WordPress password
wp = Client('https://yourdomain.com/xmlrpc.php', 'username', 'password’)
Setting Up The Post Title And Category
After authentication, the next lines of code create a WordPress post object and sets the title and category.
This creates the post object:
post = WordPressPost()
Note that this isn’t creating a post on WordPress. You are just creating an object in Python.
Post Title
The next line sets the title.
Note that when you set the title, you don’t need to start with a H1 in your content (we’ll get to the content later). Otherwise, you’ll end up with two H1s in the post.
post.title = f'Your Post Title'
Post Category
You can set both categories and tags, but I don’t use tags.
This line sets the category of the post. If you don’t set one, then WordPress puts it into the default category.
post.terms_names = {'category': ['name-of-category']}
Create A Post In WordPress Using Python
Now that you’ve got a post title and category, you have the bare minimum to create a post on your WordPress installation.
Actually, you don’t even need to set the category!
But wait, what about the content? I’ll get to that in the next section.
At this point, we’ll create a bare-bones post. This is all that you need:
post.id = wp.call(NewPost(post))
I established the variable “wp” earlier as the authenticated object. The NewPost() function adds the post to your WordPress website.
Each post has a unique ID, which is returned by the function.
If you log into your WordPress dashboard manually and refresh the “All Posts” tab, you should see a new post in draft mode.
Draft or published post?
I deliberately left the post in draft mode. You can choose to publish the post immediately, but that may not be advisable when you’re trying out the code.
But what if you want to publish it? Add these two lines after the NewPost() call. The EditPost() function requires the unique post ID, which is why I grabbed it earlier.
post.post_status = 'publish'
wp.call(posts.EditPost(post.id, post))
Writing Simple Content With Python
The simple examples I found online had a single line of text to form the content.
It would be something like this before you call the NewPost() function above.
post.content = 'Hello World.'
post.id = wp.call(NewPost(post))
This kind of example left me wondering how to deal with subheadings (H2/H3) and other elements. Read on for a clearer explanation.
Example Of Creating Realistic WP Posts With Python
There are several ways to go about this, but here is what was easiest for me.
When setting the content attribute of the post object, I built it up as HTML.
Here’s an example of building up a string variable:
wpcontent = f‘<p>This is my opening paragraph.</p>’
wpcontent += f‘<h2>First Heading</h2>’
wpcontent += f‘<p>This is a paragraph after the first H2.</p>’
wpcontent += f‘<p>This is a second paragraph after the first H2.</p>’
Once you’ve got all your content ready, then set the attribute and create the post:
post.content = wpcontent
post.id = wp.call(NewPost(post))
Putting It All Together: Complete Code
Here is a realistic Python script that creates a new post on WordPress.
from wordpress_xmlrpc import Client, WordPressPost
from wordpress_xmlrpc.methods.posts import NewPost
from wordpress_xmlrpc.methods import posts
def create_content():
wpcontent = f‘<p>This is my opening paragraph. Blah blah blah. </p>’
wpcontent += f‘<h2>First Heading</h2>’
wpcontent += f‘<p>This is a paragraph after the first H2.</p>’
wpcontent += f‘<p>This is a second paragraph after the first H2.</p>’
return wpcontent
def new_post(wpcontent):
wp = Client('https://yourdomain.com/xmlrpc.php', 'username', 'password')
post = WordPressPost()
post.title = f'My Post Title'
post.content = wpcontent
post.terms_names = {'category': ['post-category']}
post.id = wp.call(NewPost(post))
if __name__ == '__main__':
wpcontent = create_content()
new_post(wpcontent)